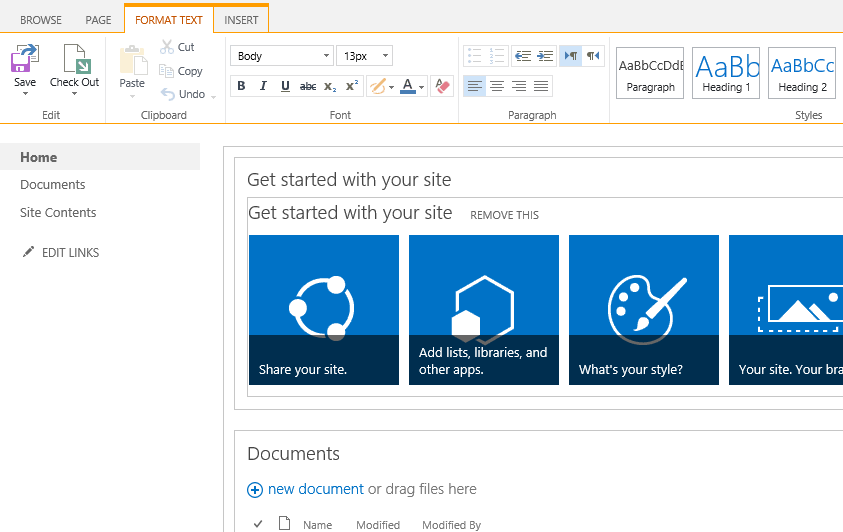
This morning I had an issue trying to implement a Phone Directory on a client’s home page. The UI incorporated a First Name and a Last Name input box and a “Go” button. But as we all know, users like to hit Enter, and we all want to try and support the best user experiences we can. However, SharePoint’s default implementation of the Enter key can sometimes put the page in edit mode…
So how do you get around this… two things..
- You need to stop the event from propagating, not that this is really the culprit but if you’re doing widget type work it’s just good practice to make sure that what you’re doing doesn’t affect the functionality of the rest of the page.
- You need to ignore the SharePoint’s default behavior of the enter key.
So what does this look like?
Let’s say you had the following DOM:
<div>
<input placeholder="First" onkeydown=" MYCODE.onEnter();" />
<input placeholder="Last" onkeydown=" MYCODE.onEnter();" />
<input style="cursor: pointer;" onclick=" MYCODE.go();" />
</div>
And the following script:
"use strict"
var MYCODE = MYCODE || {};
MYCODE.go = function () {
//Code to execute Phone Directory search goes here
}
MYCODE.onEnter = function onEnter() {
//See options below`
}
There are a few ways to accomplish the same thing:
Option 1 (Old School):
if (event.keyCode == 13) {
MYCODE.go();
event.returnValue = false;
}
Option 2 (Modern and Sexy):
if (event.keyCode == 13) {
MYCODE.go();
event.preventDefault();
event.stopPropagation();
}
Option 3: (Perfectionist)
if (event.keyCode == 13) {
MYCODE.go();
if(event.preventDefault){
event.preventDefault();
event.stopPropagation();
}else{
event.returnValue = false;
}
}
Happy Coding!